Fetch data from URLSplit each new line into a list itemSplit each line into list items- ~~Find the six-digit video ID from the URL, it should be the first integer in HTTP paths of:
c13.adrise.tv/04C0BF/v2/sources/content-owners/
c13.adrise.tv/04C0BF/ads/transcodes/
~~
Group by Video IDGet Cache Hit and Misses for each VideoCalculate the Cache Hit Misses- Sort by video ID
sort-a-list-by-integer.test.ex
import AccessLogApp.CLI, only: [
...
sort_by_field: 2
]
test "Sort by field name" do
list = [
%{:video_id => 9478, "TCP_hit_percentage" => "75%"},
%{:video_id => 234567, "TCP_hit_percentage" => "100%"},
%{:video_id => 9999, "TCP_hit_percentage" => "100%"},
%{:video_id => 004567, "TCP_hit_percentage" => "100%"},
%{:video_id => 123456, "TCP_hit_percentage" => "0%"},
%{:video_id => 009899, "TCP_hit_percentage" => "100%"},
]
result = sort_by_field(list, :video_id)
assert result == [
%{:video_id => 004567, "TCP_hit_percentage" => "100%"},
%{:video_id => 9478, "TCP_hit_percentage" => "75%"},
%{:video_id => 009899, "TCP_hit_percentage" => "100%"},
%{:video_id => 9999, "TCP_hit_percentage" => "100%"},
%{:video_id => 123456, "TCP_hit_percentage" => "0%"},
%{:video_id => 234567, "TCP_hit_percentage" => "100%"}
]
end
sort-a-list-by-integer.ex
def group_by_id(list) do
Enum.group_by(list, fn [video_id, _] ->
[video_id]
end)
end
Launch Your Project
Get your project off the ground
with Space-Rocket!
Fill out the form below to get started.
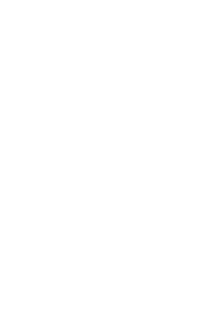